Applying JSON Patch Operations with ASP.NET Core Web API
APIs have become indispensable to modern digital businesses, not least because of their flexibility and ease of integration.
At Catchpoint, we continually strive to keep our systems updated with the latest technology & trends, including the ability to expose different APIs so that developers can use them to perform a wide variety of operations. We are excited to be soon launching our latest version of the REST API where we will be not only achieving parity with the existing version but adding new features and technical enhancements for developers dealing with APIs. One visible change to the REST API is connected to Patch operations, which can now be used for updating an entity (previously, the POST operation was the pathway for users to update entities).
In this blog, we will demonstrate how to use JSON Patch to update an entity and help you identify any issues during implementation.
This quick guide on Patch Operations covers:
- Introduction to JSON Patch
- Operations that JSON Patch supports
- Different use cases
- Key takeaways
Introduction to JSON Patch
Many APIs use JSON as their base content type (requests & responses). JSON is an open standard of attribute key/value pairs that is widely used in programming, and commonly for HTTP requests and responses, for example, JSON can be used for a range of operations, including POST, and PATCH.
Our API accepts standard JSON in the request body to specify updates for a resource. A typical JSON document may have different types of attributes, for example, simple attributes of string types, object types or array/list types. These attributes get updated according to different business needs. Operations involved might include adding a new object in an Array/List, removing an object, updating it completely or partially. There are similar operations for other types, such as objects/strings. A JSON Patch Document can specifically be used in (Rest API) Patch Operations, in which a user may want to add/replace/remove part of the (existing) JSON document (a business entity).
The Newton soft JSON Patch Document NuGet package (Microsoft.AspNetCore.Mvc.NewtonsoftJSON) supports different operations for different scenarios. However, in some instances, it behaves differently – which we will cover.
Developer prerequisites/assumptions
A basic understanding of Rest API paradigms, .Net Core and C#.
The problem
Typically, developers must deal with a range of different types of JSON document and apply a variety of Patch operations when doing so. JSON Patch Document operations behave differently with different data types. This article attempts to demonstrate how you can simplify handling different operations with JSON Patch Documents, along with highlighting some of its limitations.
We will look at how to apply a range of different Patch Operations on a range of object types, such as:
- Plan string
- Object
- Array of objects
Quick code setup for a demo application
The .Net core solution “PatchSamples.sln” has an API project and an API.Test project. Download the patch examples (zip).
PatchSampleController has different patch methods. The model Animal represents the animal.JSON document below. We will take a complex JSON document to demonstrate a series of different operations.
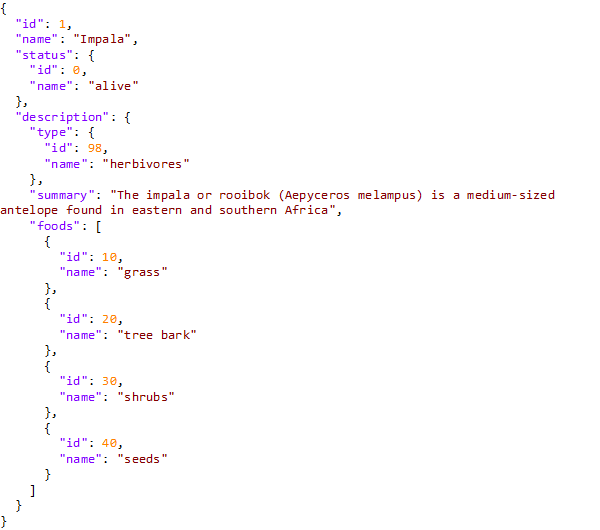
Operations that JSON Patch supports
JSON Patch is a format for specifying updates to be applied to a resource. A JSON Patch document has an array of operations. Each operation identifies a particular type of change. Examples of such changes include adding an array element or replacing a property value. (as defined @Microsoft Documentation).
Before exploring different use cases, it is important to understand the JSON Patch Document request schema. The key properties are as follows:
The root of the schema is a JSON Array, which contains a list of complex objects called an operation. The operation object has the following key properties:
- “OP” – Operation allowed – Add, Replace, Move, Copy etc.
- “PATH” – Used to specify an element or a property. It always starts with “/” (root), for example, for the attached sample: JSON.JSON for Name property, the path will be /Name
- “VALUE” – The value can be a string for a string property, an element for an array or an element type of property.
Within a JSON Patch document, a single request can have multiple operations.
Different use cases
Now let's look at some different possible use cases, starting with:
The add operation
This includes:
String add operation
The JSON Patch Document request to add a “name” property needs a value, “New Name”:


Object add operation
The JSON Patch document request to add an object as a value to “animalType” property:

If the property is a child of another object, then a parent of that object must be initialized. In the above example, if the “description” is null, the operation will fail and throw this error:

Object add operation in a list case (1)
The JSON Patch document request to add an object at the end of an already initialized or pre-populated Array/List type of property:
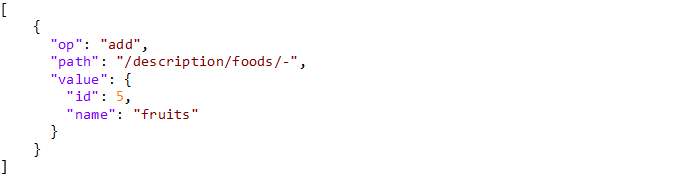

Object add operation in a list case (2)
The JSON Patch Document request to add an Array/List type of property, which is not initialized or pre-populated.
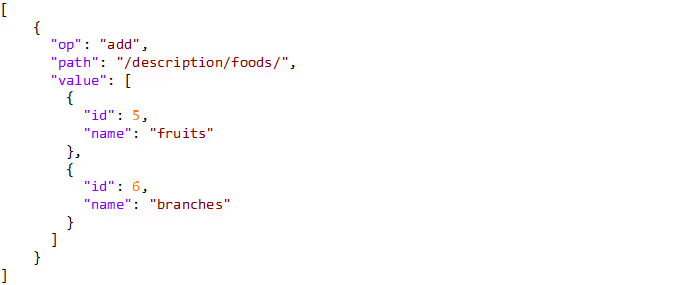

The remove operation
Remove operations include:
String remove operation
This will remove the property value on the specified path, if there is no value or if the value is already null, it won’t throw any errors:


The path should exist in the document you want to apply a patch to, otherwise it will throw an error:


Object/Element remove from list operation
The request below will remove an item from an Array/List type of element of the Patch document:


The replace operation
The Replace operation is actually a series of two operations: “Remove” & “Add”. This operation will remove the value specified on the path and then add the new value to it. Therefore, you can relatively request only an add operation:
String & object replace operation


Object replace operation in a list
The below request with “-” (hyphen) at the end of the path will remove the last element of a List. The hyphen can be replaced by an index value for a specific element:

Array replace operation in a list
To replace an Array/List property with another one:
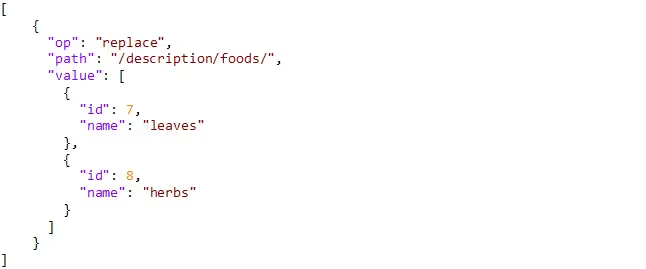

The move/copy operation
Move/Copy has the same type of request and acts similarly except for one operation:
- Move – Copy the value from the “From” location to “Path” location and apply a remove to “From” location.
- Copy – Copy the value from the “From” location to “Path” location.


The test operation
This is the test used to check the “value” specified at the “path” field. If the check succeeds, it will return “200 OK”. Otherwise, it will return, “400 Bad Request”. This operation can be used before updating any filed ones.
This operation is useful when clubbed with multiple operations to check a condition before executing the next operation:


The multiple operation
There can be multiple operations in a single request, and they can all be of different types.
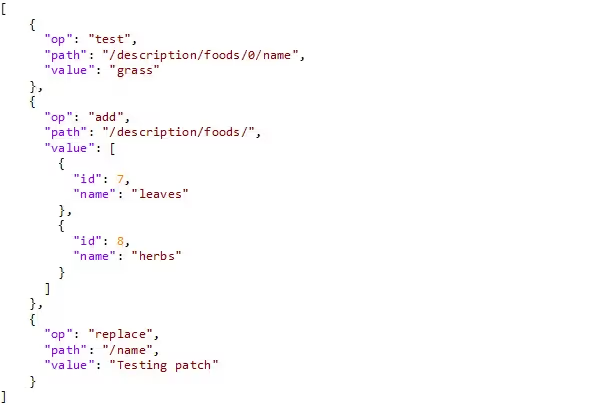

Operation control
The JSON Patch NuGet provides a list of supported operations that can be used to allow specific operations that an API endpoint can support. It can also be extended and mapped with other roles (through some customization):

You need to reference an assembly for OperationType - Microsoft.AspNetCore.JSON Patch.
Uses of JSON Patch document with dynamic objects
You can use JSON Patch document with dynamic objects as well:

You can create an instance of the JSON Patch Document and all the operations that we have discussed so far as methods of this class.
Other uses of JSON Patch document
The JSON Patch doument is also handy when it comes to changing a JSON document.
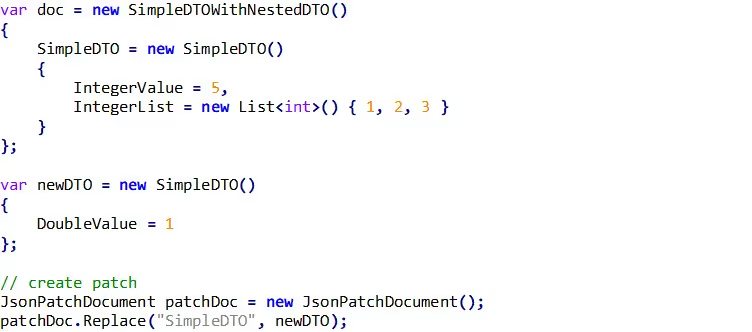
Or:

Key takeaways
- You can update the resource without knowing other values of the object. As an API consumer, I do not need to send an entire object as a payload for updating only a single property.
- I can make different operations (add/remove/replace) perform on a property/attribute. You don’t need to have different methods for modification and call them separately.
- Another plus is that you can call multiple operations on different properties/attributes in a single Patch call. Thus, a single request can handle several different needs.
- Finally, the new REST API will reduce the payload required to make an update call by sending it over the wire.
I hope if you are new to HTTP Patch, this blog has helped you gain a foundational knowledge base around JSON Patch and if you are already using it, it will deepen and refine your technical knowhow.