API architecture refers to the design and structure of Application Programming Interfaces, specifying how different API components should interact and communicate. The API architecture consists of rules that determine functionalities to provide to the API consumers.
This article delves into the essence of API architecture and its components, common types of API architectures, and key practices to develop better APIs.
Summary of key API architecture concepts
What is API architecture?
An application programming interface (API) is a software interface that exposes backend data and application functionality for use in new applications. API architecture is a set of rules and common practices that define how an API interacts with other components. It provides a framework for designing, developing, and delivering backend services. With the right API architecture, you can create applications that are modular and reusable.
{{banner-32="/design/banners"}}
Overview
You can divide API architecture into four primary layers.

Data layer
It is responsible for storing, retrieving, and manipulating data. It typically includes databases, data storage systems, and persistent data components. The primary role of the data layer is to ensure that information is stored and retrieved efficiently.
Integration layer
The integration layer enhances interoperability by managing the integration of various systems and services. It sits between the data layer and the application layer, facilitating communication and coordination of data. It also plays a vital role in tasks such as data transformation, validation, and ensuring continuous information flow between different components.
Application layer
The heart of the API architecture resides in this layer. It defines how the API functions and what operations it can perform based on the received requests. It hosts the business logic and functionality, interpreting and processing incoming requests. It also executes the necessary operations and orchestrates the overall behavior of the API.
Interaction layer
The interaction layer serves as the interface between the API and external systems, applications, or users. It manages incoming requests, handles authentication, and communicates responses. As the gateway for API interactions, it provides security and enhances efficiency in communication with the outside world.
Components
We explain the API architecture components in the table below.
{{banner-31="/design/banners"}}
Common API architecture types
Different API architecture types exist to meet varied functionalities and performance requirements. Some styles emerged to better support changes in communication protocols due to technology advances. Some styles focus on speed and efficiency, like gRPC, while others prioritize data integrity over performance. We explain common styles below.
SOAP
Simple object access protocol(SOAP) maintained by the World Wide Web Consortium (W3C), is one of the earliest API architectures. It utilizes XML for message formatting, ensuring a standardized approach to communication between applications.
In SOAP terminology, the client is the service requester, and the server is the service provider. The exchange XML message is in a standardized structure with an "Envelope" element, typically containing a "Header" and "Body" section. The web service description language (WSDL) defines SOAP APIs, offering a comprehensive blueprint for their structure.
While REST focuses on lightweight interactions, SOAP prioritizes structured data exchange and robust security. SOAP APIs excel in scenarios where security, consistency, and durability are paramount. However, being older technology, SOAP APIs are less popular in a modern microservices architecture.

REST
APIs built on the representational state transfer (REST) architecture are popular among developers due to their simplicity. RESTful APIs follow six architectural principles.
REST APIs adhere to six key principles that guide their design and functionality:
- Separation—of the client (application requesting data) and the API server (providing data).
- Statelessness—Each request sent by the client to the server must contain all the information needed for processing.
- Resource-based—REST APIs present data and functionality as resources accessible through URIs.
- Uniform Interface—Consistent rules for how clients interact with server resources.
- Cacheable—Responses to requests should be cacheable by both the client and server as much as possible.
- Layered system—The architecture allows for the presence of intermediaries like caches and load balancers between servers and clients
To access and manipulate resources, clients can use standard HTTP methods such as GET, POST, PUT, and DELETE. Every resource has a unique identifier for efficiency. The use of headers and parameters in requests enhances the flexibility for developers.
REST APIs are preferred because they are easy to scale. More server resources or intermediaries can be added or removed flexibly if client requests increase. Well-defined principles simplify maintenance and upgrades.
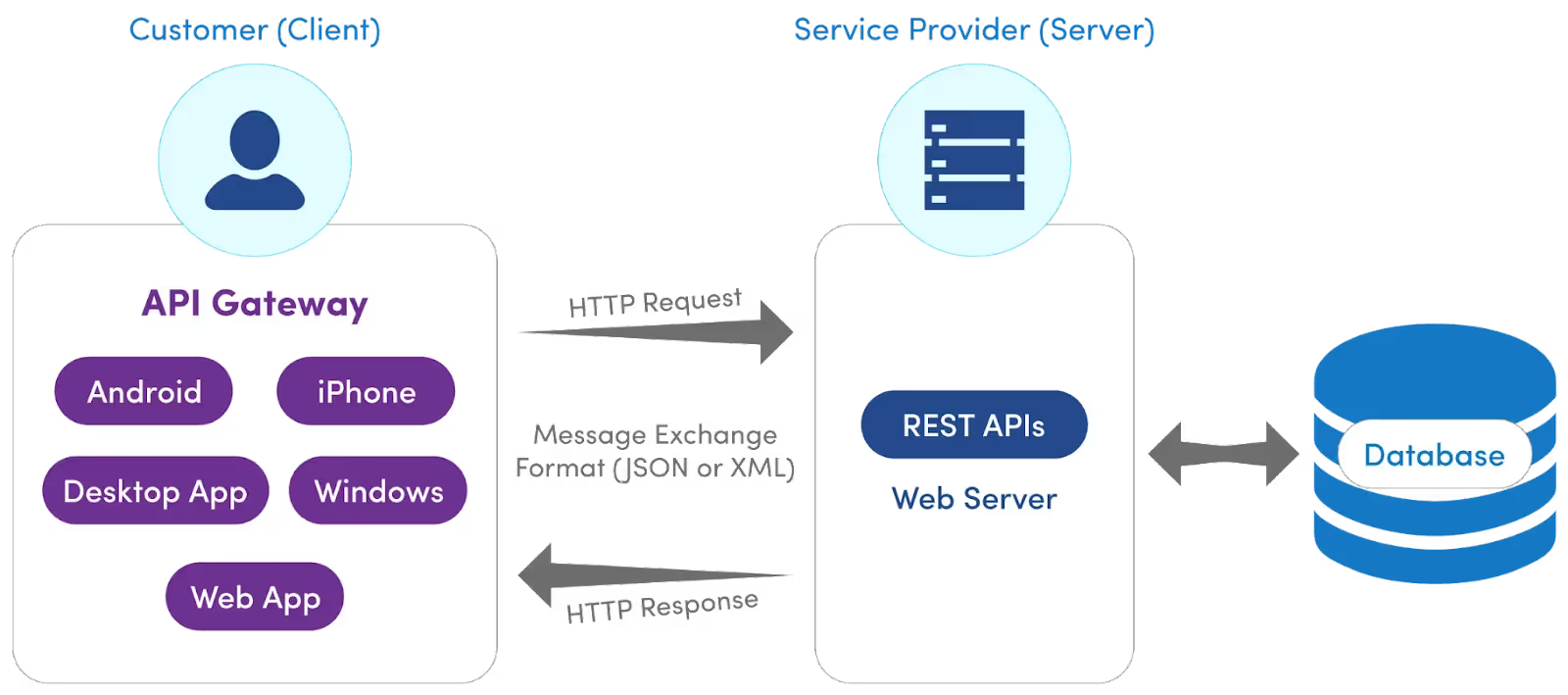
gRPC
gRPC, developed by Google, is an API architecture style that uses remote procedure calls for API interaction. Services expose functionalities as methods; clients can call them similar to local function calls.
gRPC employs Protocol Buffers, a language-neutral format for defining data structures and messages. It enables efficient communication between diverse programming languages. Like REST, it supports single request, single response communication, but being a more modern API style, it also has built-in support for server-streaming (continuous server updates), client-streaming (sending a stream of data), and bidirectional streaming (real-time communication).
gRPC prioritizes fast and efficient data exchange, making it ideal for latency-sensitive applications. Developers appreciate its extensibility and speed, making it suitable for scenarios demanding rapid and efficient data exchange.

WebSocket
A WebSocket is a communication protocol that enables full-duplex, real-time communication between a client and a server. Unlike traditional HTTP requests, WebSockets enable bi-directional communication, allowing both client and server to initiate and receive messages as needed. This facilitates real-time data exchange and interactive experiences.
Instead of opening and closing connections for each message, WebSocket APIs establish a single, persistent connection over which data flows continuously. Data is typically exchanged in JSON or Protocol Buffers to optimize transmission speed. This reduces network overheads and improves communication efficiency.
Unlike traditional request-response architectures, WebSocket APIs facilitate instant data transfer between clients and servers. This makes them an excellent choice for applications requiring low-latency updates, such as live dashboards, chat, online gaming or financial trading platforms.
GraphQL
REST APIs supported limited granularity in data exchange between APIs. For example, if customer data is requested, REST APIs return the complete server record, and the client filters the data it needs from the server. This sometimes results in overfetching or underfetching data, leading to inefficient communication. In contrast, GraphQL architecture allows clients to specify the exact data they need. This is achieved through queries written in a flexible language like GraphQL Schema Language (SDL).
The API functionality is defined by a schema that describes available data types, fields, and their relationships. Clients use the schema to generate queries. Clients can request nested data structures in a single query, retrieving related data in one go. This simplifies data fetching and eliminates the need for multiple server calls.
GraphQL queries are declarative, specifying the data needed but not how it's retrieved. This allows the server to optimize execution and leverage different data sources efficiently. GraphQL also provides a structured error-handling mechanism that returns detailed information about errors encountered during query execution.
You can consider using GraphQL when the client needs are diverse and dynamic, complex data structures and data relationships exist, and network resources are limited.
{{banner-30="/design/banners"}}
Key practices to build better APIs
You can follow the below practices to develop more efficient API architectures.
Versioning
Implementing versioning ensures backward compatibility and smooth transitions when introducing changes to the API. It allows for the evolution of the API without disrupting existing consumers, fostering a more reliable and adaptable ecosystem.
Consistent naming
Maintaining uniform and accurate names for resources and endpoints enhances clarity and predictability. Consistency in naming conventions simplifies understanding, making the API more user-friendly for developers.
Resource grouping
Organize resources logically by grouping related functionalities. It improves the API's structure and enhances the developer experience by providing a coherent and intuitive arrangement of features.
Monitoring
Leverage API testing and monitoring tools. Catchpoint provides an excellent set of tools that helps to gain insights into API performance. Set up comprehensive monitoring, alerting, and reporting mechanisms to proactively identify and address issues, ensuring optimal availability and responsiveness. Also, use transparent HTTP status codes and properly articulate messages in API responses to enhance communication efficiency between clients and servers. This practice promotes better understanding and handling of requests, contributing to a more streamlined and reliable interaction within the API.
Caching
Implement an effective caching strategy to reduce response times and minimize server load. Utilize caching mechanisms intelligently to store and serve frequently requested data, improving overall API efficiency. Also, pay extra attention to do it sparingly with proper cache expiration.
Scalability
Design the API architecture with scalability in mind from the beginning. Employ scalable infrastructure, load balancing, and distribution strategies to accommodate increasing demand while maintaining performance and responsiveness.
Security
Prioritize security by implementing robust authentication and authorization mechanisms. Enforce encryption for data in transit, handle sensitive information securely, and stay updated on security best practices to protect against potential vulnerabilities.
Documentation
Thoroughly document the API, providing clear and comprehensive information on endpoints, request/response formats, authentication methods, and usage examples. Well-documented APIs ease integration efforts and enhance developer satisfaction.
Error handling
Implement robust error-handling mechanisms to provide meaningful error messages and status codes. Clear and informative error responses aid developers in troubleshooting and resolving issues promptly, improving the overall user experience.
Testing and validation
Implement adequate tests to ensure the reliability of the API endpoints. Also, perform load testing and proper validation on all the endpoints exploring various loads and scenarios using Catchpoint's comprehensive testing tools to keep the API healthy and resilient. This approach helps to identify potential issues early, guaranteeing optimal performance and responsiveness.
Last thoughts
API architecture serves as the foundation for software integration, modularity and reusability. This article explored its components, layers, types, and best practices for optimal API development. Understanding the modular structure of API components and layers is crucial, emphasizing the need for a cohesive framework. The varied types, from RESTful to GraphQL, showcase the adaptability of API architectures to diverse application requirements.
Key takeaways include the importance of simplicity, adherence to security standards, and comprehensive documentation for robust API development. A well-crafted API architecture in an evolving digital landscape remains pivotal, enabling efficient communication and collaboration between systems.
{{banner-28="/design/banners"}}